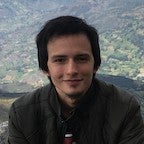
MinIO is a highly-available, S3 compatible object storage solution. It can be used with Node.js via either the MinIO SDK or the AWS SDK. Deploy a managed MinIO cluster on Northflank. The following guide will focus on providing basic scripts for communicating to your MinIO instance over TLS using the MinIO SDK.
At the end of this guide you will be able to create a Node.js project, connect it to your MinIO instance and:
- Create and list buckets
- Create objects, upload files and list object keys
- Read objects and store them as files
- Node.js
- npm
- MinIO server running with TLS
node-with-minio/
├─ minio-connect.js
├─ minio-create-buckets.js
├─ minio-create-objects.js
├─ minio-read-objects.js
├─ .env
└─ package.json
The full source code used in this guide can be found in this git repository
npm install minio
npm install dotenv
Create a file named .env
which will contain all the credentials and details for connecting to your MinIO instance, such as HOST
, ENDPOINT
, ACCESS_KEY
and SECRET_KEY
.
We’ll use the dotenv
package to load these values as environment variables when the project starts. The https
prefix used on the ENDPOINT
environment variable will make sure your connection is established with TLS.
HOST=<host>
ENDPOINT=https://<host>:<port>
ACCESS_KEY=<value>
SECRET_KEY=<value>
In order to connect to your MinIO instance, the first step is to import the dotenv
package and call the config()
method, which will load the configuration as environment variables. Also keep in mind the pathStyle: true
option, required in case MinIO doesn’t support virtual-host
style URLs. The next step is to create the MinIO client. Make sure to provide the proper credentials and endpoint as shown in the code snippet below.
require("dotenv").config();
const Minio = require("minio");
const minioClient = new Minio.Client({
accessKey: process.env.ACCESS_KEY,
secretKey: process.env.SECRET_KEY,
endPoint: process.env.HOST,
pathStyle: true,
});
Run this script from your terminal with node minio-connect.js
The next step after instantiating the MinIO client is to create and list buckets.
Buckets represent the way MinIO server arranges the data. All you need to do to create one is supply the name you'd like the bucket to have. Buckets contain a list of objects and these represent the data stored on the server. Objects are identified by a key and also contain system and user metadata.
The following snippet creates a bucket named “second-bucket” using the makeBucket
method from the MinIO client. Then listBuckets
is used to fetch buckets owned by your account.
const bucketName = "second-bucket";
(async () => {
console.log(`Creating Bucket: ${bucketName}`);
await minioClient.makeBucket(bucketName, "hello-there").catch((e) => {
console.log(
`Error while creating bucket '${bucketName}': ${e.message}`
);
});
console.log(`Listing all buckets...`);
const bucketsList = await minioClient.listBuckets();
console.log(
`Buckets List: ${bucketsList.map((bucket) => bucket.name).join(",\t")}`
);
})();
The following snippet creates an object named “file.txt” on the “second-bucket” bucket you just created. The data of the object is provided on the body argument which corresponds to the string “Hello There!”. The method putObject
from the MinIO SDK is used to submit the object's data to the MinIO server.
Create or update the script minio-create-object.js
and run it with node minio-create-buckets.js
.
const bucketName = "second-bucket";
(async () => {
// create object with string data
const objectName = "file.txt";
const result = await minioClient
.putObject(bucketName, objectName, "Hello There!")
.catch((e) => {
console.log("Error while creating object: ", e);
throw e;
});
console.log("Object uploaded successfully: ", result);
})();
In this case the object’s data comes from a file. The file is first read using the fs
package with the readFileSync()
method. The same putObject
method is used and the values for the body argument are now the buffer from the file “file.txt” located in the same directory. In this case the object’s key is “file-object.txt” which will be stored on the “second-bucket” bucket.
Create or update the script minio-create-buckets.js
and run it with node minio-create-object.js
.
const fs = require(“fs”);
const bucketName = "second-bucket";
(async () => {
// create object from file data
const objectFileName = "file-object.txt";
const fileData = fs.readFileSync("./file.txt");
const submitFileDataResult = await minioClient
.putObject(bucketName, objectFileName, fileData)
.catch((e) => {
console.log("Error while creating object from file data: ", e);
throw e;
});
console.log("File data submitted successfully: ", submitFileDataResult);
})();
The getObject
method is used for fetching a object’s data. The process is done in chunks, hence, we add a listener to the “data” event. This represents events where the server sends parts of the object's data. Our listener will take each chunk of data and write it on a WriteStream
object that points to the read-in-chunks.txt
file.
In order to run this sample, create a script called minio-read-objects.js
and run it with node minio-read-objects.js
.
const fs = require(“fs”);
const bucketName = "second-bucket";
(async () => {
// read object in chunks and store it as a file
const fileStream = fs.createWriteStream("./read-in-chunks.txt");
const fileObjectKey = "file-object.txt";
const object = await minioClient.getObject(bucketName, fileObjectKey);
object.on("data", (chunk) => fileStream.write(chunk));
object.on("end", () => console.log(`Reading ${fileObjectKey} finished`));
})();
In this how-to guide we have shown how to use Node.js to connect to a MinIO instance using the MinIO SDK, how to create buckets and how to read and write objects in those buckets. The full source code used in this guide can be found in this git repository.
In the first step, we create an .env
file which contains the URI connection string to MinIO and connect the MinIO instance using the MinIO SDK. Once the MinIO instance is set up, we created and listed buckets. In the final step we uploaded objects as files and read and wrote objects from files.
Northflank allows you to spin up a MinIO database and a Node.js service within minutes. Sign up for a Northflank account and create a free project to get started.
- Multiple read and write replicas
- Observe & monitor with real-time metrics & logs
- Low latency and high performance
- Backup, restore and fork databases
- Private and optional public load balancing as well as Northflank local proxy