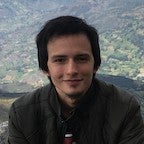
MongoDB is a NoSQL document-based database. It supports different deployments for high-availability and scalability use cases. This guide introduces you to connecting your Node.js application to a MongoDB database and how to read and write data.
At the end of this guide you will be able to create a Node.js project, setup a connection to a MongoDB database and read and write some data.
- Your local machine with Node.js & npm installed https://nodejs.org/
- Create a new directory and initialize an empty Node.js project with
npm init
- A running instance of MongoDB with TLS configured
node-with-mongodb/
├─ package.json
├─ .env
└─ mongodb.js
The full source code used in this guide can be found in this git repository.
In this guide, we will be using the official Node.js MongoDB module to connect to our database. We’ll also use the dotenv
package to load environment variables specified in the .env
file when the script is executed. They can be installed using npm:
npm install mongodb
npm install dotenv
All the code examples will be part of the mongodb.js
file in the project directory.
The .env
file will contain the URI connection string. This contains the user, password, host address as well as database and extra connection options for connecting to your MongoDB database.
MONGO_URI='mongo+srv://<user>:<pass>@<host>:<port>/<database>?<connection options>'
Once the .env
file is ready, in the mongodb.js
script we can load the contents of that file as environment variables:
require('dotenv').config()
This will allow us to use process.env.MONGO_URI
in our scripts.
Connecting to your database with TLS encrypts all network data between the MongoDB server and your client. This also helps to ensure the client is connected to the intended server.
The Node.js MongoDB library supports this setting. The simplest way to connect to your database is by using the URI with all the required data: user, password, database, host, port, and other options such as replica set, TLS, or authSource.
The URI should have the host and all the credentials required for connecting to the database. In case of using TLS, an extra parameter tls: true
can be provided to the MongoClient constructor.
const client = new MongoClient(uri, {
tls: true,
})
However, if you use a connection URI with mongo+srv
prefix, tls will be automatically enabled, so the tls: true
option can be omitted.
The following snippet shows the client configuration and connection to the database. It also queries the database information and prints the role of the MongoDB node.
require('dotenv').config()
const { MongoClient } = require('mongodb');
const uri = process.env.MONGO_URI;
const client = new MongoClient(uri);
(async () => {
try {
await client.connect();
const dbRole = await client.db().command({ hello: 1 });
console.log(
`Role of database - Host: ${dbRole.me} Is primary: ${dbRole.isWritablePrimary}`
);
await client.close();
} catch (e) {
console.log('Error: ', e.message);
}
})();
Now that we’re connected to our database, we can proceed to write and read data.
In order to do this, we have to use the MongoClient we created in previous steps and access a collection of documents called 'movies':
await client.connect();
const moviesCollection = await client.db().collection('movies');
console.log('Collection name: ', moviesCollection.name);
If the collection doesn’t exist it will be automatically created for us. The next step is to add document to the movies
collection:
const { insertedId } = await moviesCollection.insertOne({ name: 'Spider-Man: No Way Home', year: 2021 });
The insertedId
is an ObjectId that represents the ID of the new document we just inserted into the collection. We can then use that same ObjectId to query the collection and fetch the document:
const document = await moviesCollection.findOne({ _id: insertedId });
console.log(‘Document from db: ‘, document);
We can also fetch the number of documents part of a collection by calling the countDocuments()
method as follows:
console.log(‘Number of documents: ‘, await moviesCollection.countDocuments());
Now we can use the full script as follows, with all of the logic written in previous steps. This involves: Connecting to our database using TLS; adding entries into ‘movies’ collection; querying a document using ObjectId and getting the number of documents on the collection.
The full script would be as follows:
require('dotenv').config();
const { MongoClient } = require('mongodb');
const uri = process.env.MONGO_URI;
const client = new MongoClient(uri);
(async () => {
try {
await client.connect();
const dbRole = await client.db().command({ hello: 1 });
console.log(
`Role of database - Host: ${dbRole.me} Is primary: ${dbRole.isWritablePrimary}`
);
// Accessing ‘movies’ collection object
const moviesCollection = await client
.db()
.collection('movies');
console.log('Collection name: ', moviesCollection.collectionName);
// Inserting new document into the ‘movies’ collection
const result = await moviesCollection.insertOne({ name: 'Spider-Man: No Way Home', year: 2021 });
const { insertedId } = result;
console.log('Result: ', insertedId);
// Fetching a document based on ObjectId
const document = await moviesCollection.findOne({ _id: insertedId });
console.log('Document from db: ', document)
// Getting the number of documents in the collection.
console.log(
'Number of documents: ',
await moviesCollection.estimatedDocumentCount()
);
await client.close();
} catch (e) {
console.log('Error: ', e.message);
}
})();
In scenarios where the certificate served by the MongoDB database is not issued by a well-known certificate authority, connection using TLS will fail. As a consequence, the user must provide the certificate authority to verify the server certificate. This can be done through the ca
option on the MongoClient
class.
The following snippet shows how to provide a certificate authority for connecting with TLS to your MongoDB database. The file is read from the path provided to the readFileSync
method.
require('dotenv').config();
const { MongoClient } = require('mongodb');
const fs = require('fs');
const uri = process.env.MONGO_URI;
const client = new MongoClient(uri, {
tls: true,
ca: [fs.readFileSync('<path to certificate authority>')]
});
Then you can connect to your database as usual:
(async () => {
await client.connect();
})();
In this how-to guide, we have shown how to use Node.js to connect to a MongoDB instance and how to read and write data. The full source code used in this guide can be found in this git repository.
In the first step, we create an .env file which contains the URI connection string to MongoDB. These variables are then used to connect to a MongoDB instance. In the final step, we showed how to read and write data from our database.
Northflank allows you to spin up a MongoDB database and a Node.js service within minutes. Sign up for a Northflank account and create a free project to get started.
- Multiple read and write replicas
- Observe & monitor with real-time metrics & logs
- Low latency and high performance
- Backup, restore and fork databases
- Private and optional public load balancing as well as Northflank local proxy