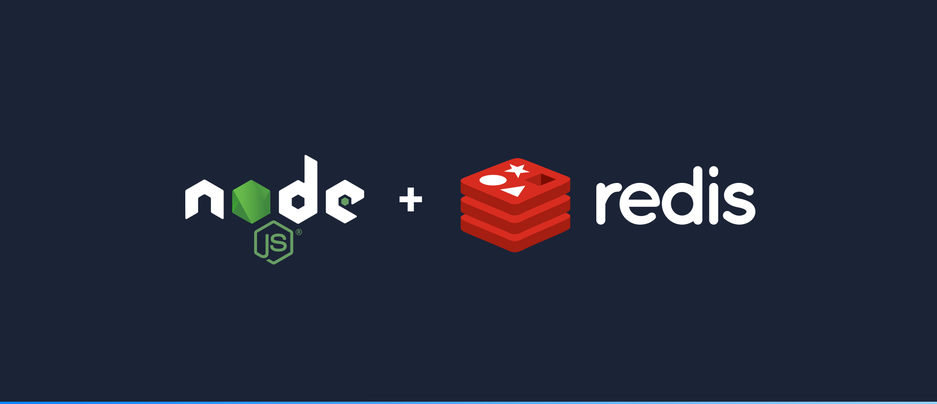
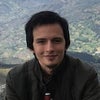
Redis is a high-performance in-memory key-value store, useful in a wide range of scenarios such as a database, cache, message broker and queue. In Node.js there are two main libraries for interacting with the Redis server: node-redis
and ioredis
. This guide introduces you to how to connect your Node.js application to a Redis database, with both libraries, and shows how to read and write data.
This guide will focus on the following use cases with both packages:
- Connect to your redis instance using TLS
- Basic write and read commands.
- Node.js & npm
- Redis server configured with tls enabled and valid certificates.
node-with-redis/
├─ redis.js
├─ .env
└─ .package.json
The full source code used in this guide can be found in this git repository.
The packages we’ll use in this guide are node-redis
v4.0 and ioredis
v4.28. The node-redis
package starting with v4 comes with promises by default, which makes the difference with the ioredis
package small in most cases when issuing commands.
The command redis@next
is recommended on node-redis which will install v4.
The dotenv
dependency will be used to load credentials as environment variables from the .env
file.
npm install dotenv
Then install the redis package you want to use.
For node-redis
use:
npm install redis@next
In case you want to use ioredis
, go with:
npm install ioredis
Redis supports TLS configuration since version 6, ensuring privacy for data sent between the server and client.
Create a file named .env
in your project directory. In this guide we’ll require the REDIS_URL that contains authentication credentials and the HOST with the domain address where the redis server is deployed.
The prefix rediss
is used on the url to indicate client libraries that tls should be used.
REDIS_URL=rediss://<username>:<password>@<host>:<port>
REDIS_HOST=<domain address redis server>
Then the first step is to load environment variables through the dotenv
dependency, by calling config()
method. This way we now have access to REDIS_URL
and REDIS_HOST
environment variables.
require(“dotenv”).config()
In most cases if the redis url provided presents the rediss://
prefix, no more options are required for connecting to the database through tls.
require(“dotenv”).config();
const { createClient } = require("redis");
const redisClient = createClient({
url: process.env.REDIS_URL,
});
But if the SNI host is required, we should pass more options to the connection.
require(“dotenv”).config()
const { createClient } = require("redis");
const redisClient = createClient({
url: process.env.REDIS_URL,
socket: {
tls: true,
servername: process.env.REDIS_HOST,
},
});
In this case the socket
object contains tls attributes: boolean tls
set to true and servername
set to REDIS_HOST
environment variable. The latter takes care of sending the SNI host for connection.
Once the client is correctly configured, we can now proceed to connect to our database.
(async () => {
// Connect to redis server
await redisClient.connect();
})();
If the REDIS_URL
contains the rediss://
prefix, in case redis is deployed with tls enabled, the code will be quite straightforward.
require(“dotenv”).config()
const Redis = require("ioredis");
const redis = new Redis(process.env.REDIS_URL);
In scenarios where the client must send the SNI host, we need to provide more connection options.
const redis = new Redis(process.env.REDIS_URL, {
tls: {
servername: process.env.REDIS_HOST,
},
});
In this case the servername
attribute represents the SNI host to present during the connection with REDIS_HOST as the value. The redis client will connect when the script is executed.
The following snippets will contain different redis commands such as: ping
, get
, incr
and set
. Given that ioredis
and node-redis
are very similar to each other, there won’t be major differences when running these functions, hence, the snippets are fully compatible between both clients.
The ping
command allows us to check if the connection was successfully established and that the server can run commands.
const pingCommandResult = await redis.ping();
console.log(“Ping command result: “, pingCommandResult);
In order to fetch values for certain keys we can use the get
method passing the key
string argument. On the following snippet we first fetch the current value for the count
key, we increase it by one and then we fetch the key’s value one more time.
const getCountResult = await redis.get("count");
console.log("Get count result: ", getCountResult);
const incrCountResult = await redis.incr("count");
console.log("Increase count result: ", incrCountResult);
const newGetCountResult = await redis.get("count");
console.log("New get count result: ", newGetCountResult);
We can also set string values through the set
command, which expects the key
and value
. In this case the value
is a JSON which represents an object with name
and lastname
attributes.
await redis.set(
"object",
JSON.stringify({
name: "Redis",
lastname: "Client",
})
);
Then we can fetch the string and parse it into an object as follows:
const getStringResult = await redis.get("object");
console.log("Get string result: ", JSON.parse(getStringResult));
This is the full script with all the code provided so far. Consider which package you want to use as a redis client and if TLS is required, since connection details will differ.
The full script would be as follows:
require(“dotenv”).config()
// if using node-redis package
const { createClient } = require("redis");
const redis = createClient({
url: process.env.REDIS_URL,
socket: {
tls: true,
servername: process.env.REDIS_HOST,
},
});
// if using ioredis package
const Redis = require("ioredis");
const redis = new Redis(process.env.REDIS_URL, {
tls: {
servername: process.env.REDIS_HOST,
},
});
(async () => {
await redis.connect(); // if using node-redis client.
const pingCommandResult = await redis.ping();
console.log("Ping command result: ", pingCommandResult);
const getCountResult = await redis.get("count");
console.log("Get count result: ", getCountResult);
const incrCountResult = await redis.incr("count");
console.log("Increase count result: ", incrCountResult);
const newGetCountResult = await redis.get("count");
console.log("New get count result: ", newGetCountResult);
await redis.set(
"object",
JSON.stringify({
name: "Redis",
lastname: "Client",
})
);
const getStringResult = await redis.get("object");
console.log("Get string result: ", JSON.parse(getStringResult));
})();
In this how-to guide, we have shown how to use Node.js to connect to a Redis instance. Initially we connected with TLS using varying Node.js packages such as node-redis
and ioredis
. Then we learned how to ping, read and write data to the Redis instance.
The full source code used in this guide can be found in this git repository.
Northflank allows you to spin up a Redis database and a Node.js service within minutes. Sign up for a Northflank account and create a free project to get started.
- Multiple read and write replicas
- Observe & monitor with real-time metrics & logs
- Low latency and high performance
- Backup, restore and fork databases
- Private and optional public load balancing as well as Northflank local proxy